As given by the fast-paced world of web development, an optimal Node.js application should improve performance and simplify the deployment process. Nginx is very strong software that acts like both reverse proxy and a load balancer, which plays a very important role in improving the scalability as well as security of web applications. In this article we’ll go through setting up a Node.js app in Docker containers, configuring Nginx for load balancing and reverse proxying
What is Nginx?
Nginx is an open-source web server and reverse proxy, also often used as a load balancer. Its lightweight architecture and its ability to process multiple requests at the same time make it very suitable for high-traffic applications. Here’s why you should use Nginx:
Reverse Proxy: Nginx acts as a reverse proxy by forwarding client requests to a backend server, improving performance by offloading tasks like SSL termination and caching.
Load Balancer: Nginx distributes incoming requests across multiple instances of a server, so one instance will not get overwhelmed, thus utilizing all resources properly.
Setting Up a Node.js Application with Docker
First we setup a Node.js project
- Install Node.js: Go to https://nodejs.org/en to install Node.js. Once the Node.js is Installed, it can be verified with node -v or npm -v
- Initialize a New Project: Go to the directory of your choice; Open then terminal and run this command: mkdir demo-app; cd demo-app; npm init -y
- Create Project Files: Create file with these commands: touch app.js
- Now we will install the express package in our project setup: npm install express
- An implementation is mention below. once every thing set. you can verify the setup running project with command: node app.js
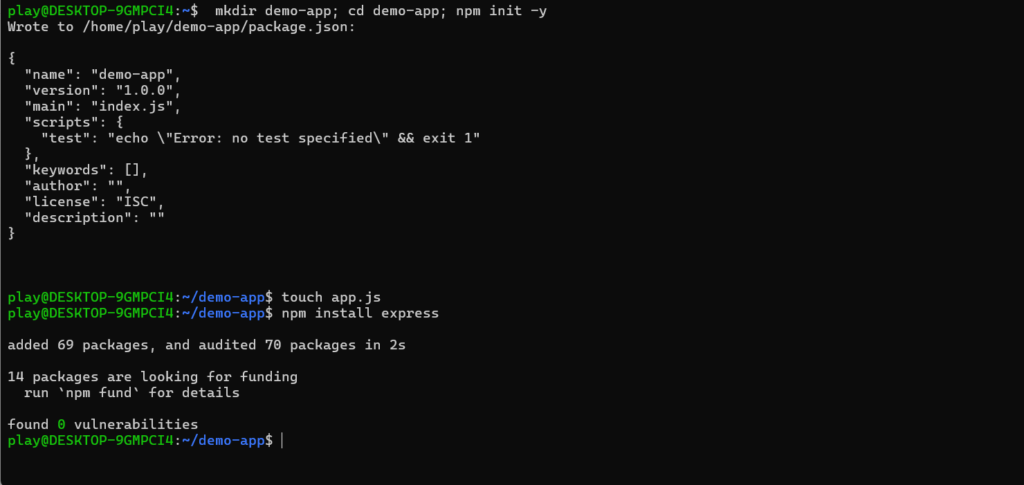
// app.js
const express = require('express');
const app = express();
const port = process.env.PORT || 3000;
const appName = process.env.APP_NAME || 'NA';
app.get('/', (req, res) => {
res.send(`Hello, this project is ${appName}`);
});
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Dockerize the Application
Create a Dockerfile
in the Node.js application setup that specifies how to build the Docker image. To create file you can run this command: touch Dockerfile
;
# Dockerfile
FROM node:22
WORKDIR /demo-app
COPY app.js .
COPY package.json .
RUN npm install
EXPOSE 3000
CMD [ "node", "app.js"]
Once the configuration is done for docker file, we can build the image for the application.
# Command to create the image
docker build -t demo-app:1.0 .
# Command to view the images list
docker images
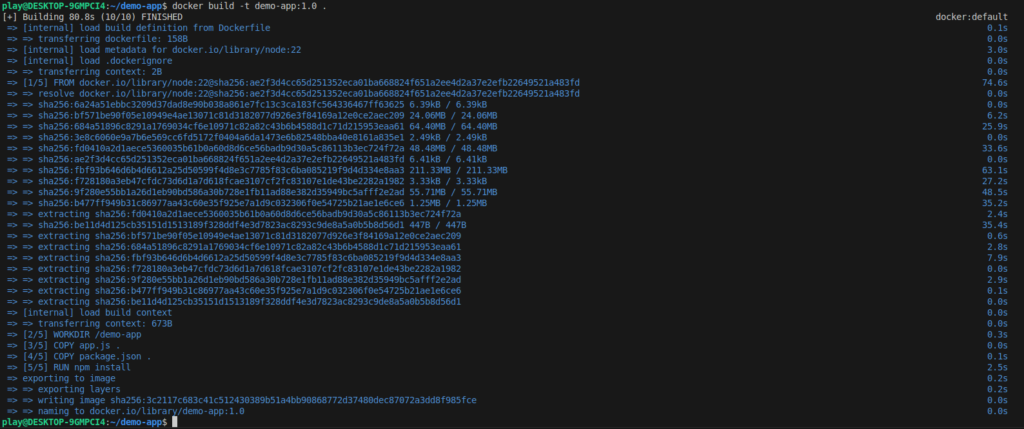
# Once image is created, we can run the project with docker image with below command.
docker run -p 3000:3000 demo-app:1.0
# demo-app:1.0 is name of the image

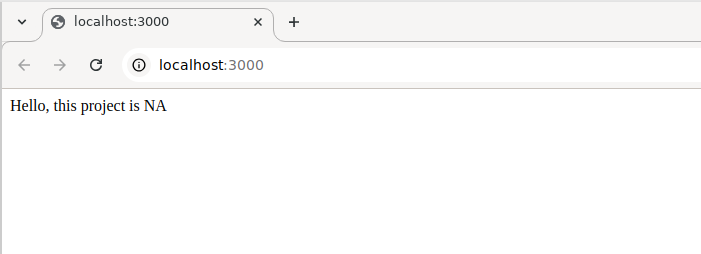
Ignore this NA for now, It is a default value for env variable
Now that our Node.js app is running with docker container. Now we will setup docker-compose.yml file to run multiple instances to demonstrate the load balancing and reverse proxy. So, create yml file by this command in the node project root directory: touch docker-compose.yml
// docker-compose.yml file configuration
version: '3'
services:
app_one:
build: .
environment:
- APP_NAME=First App
ports:
- "3001:3000"
app_two:
build: .
environment:
- APP_NAME=Second App
ports:
- "3002:3000"
app_three:
build: .
environment:
- APP_NAME=Third App
ports:
- "3003:3000"
Once the docker-compose file configuration is setup, we can run the docker compose up command to run 3 instance of the same application
# This command run services( 3 instances in our case)
docker-compose up --build -d
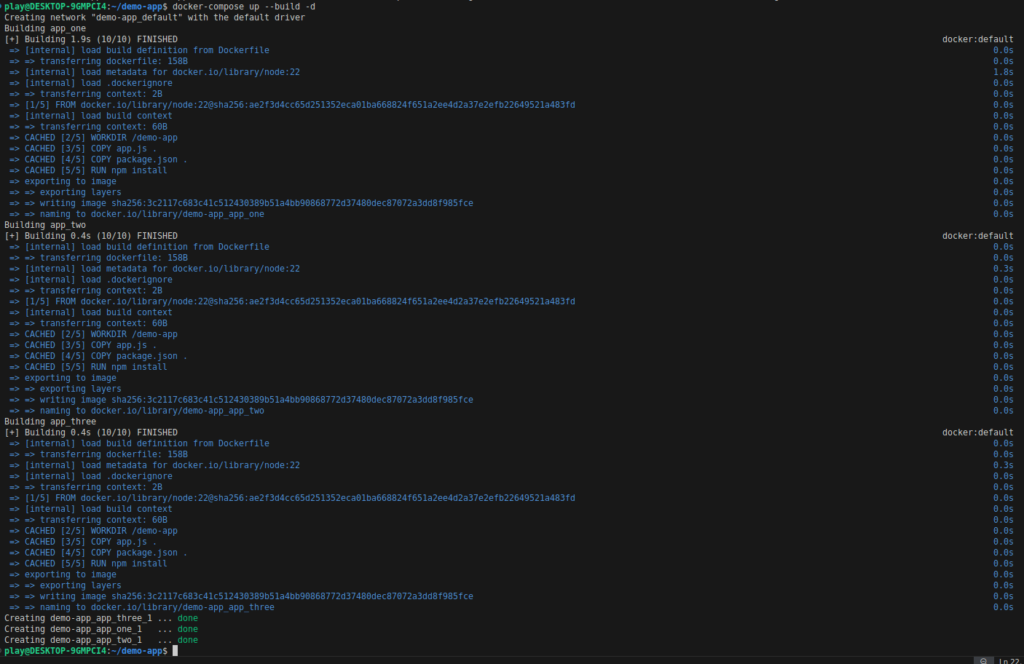
Configuring Nginx for Load Balancing and Reverse Proxy
Now that our Node.js app is containerized, we can configure Nginx to handle incoming requests and forward them to our app. We’ll also set up load balancing to distribute the traffic evenly across multiple instances of the app.
Steps:
- Install Nginx on your server.
- Set up Reverse Proxy: Configure Nginx to forward client requests to your Node.js app containers.
- Enable Load Balancing: Define multiple upstream servers in the Nginx configuration to balance the load.
Once we have nginx installed in the system, we will configure it as per our requirement: open nginx config file: nano /etc/nginx/nginx.conf
worker_processes 1;
events {
worker_connections 1024;
}
http {
upstream demo_app{
least_conn;
server 127.0.0.1:3001;
server 127.0.0.1:3002;
server 127.0.0.1:3003;
}
server {
listen 8080;
server_name localhost;
location / {
proxy_pass http://demo_app;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
}
Now that everything is setup. you can view the page in the browser and with continue refresh you will observe that requests are being redirected to different instances.
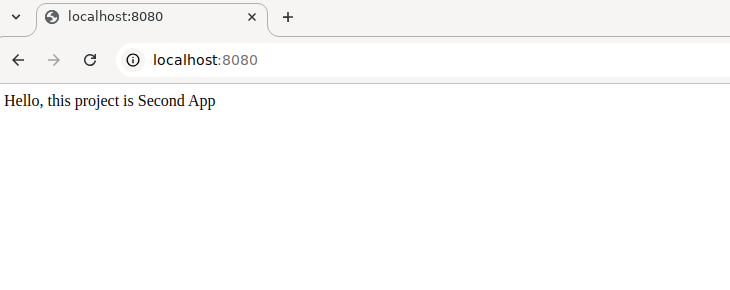
Docker Compose: Simplifying Multi-Container Management
Docker Compose is a tool to make managing applications with multiple containers in Docker more easy. Here, we are going to manage our Node.js app and the Nginx reverse proxy using Docker Compose.
Steps:
- Define Services: Use a
docker-compose.yml
file to define both the Node.js app and Nginx as services. - Scale Containers: Easily scale your application by adjusting the number of Node.js app instances.